Implementing C model integration using DPI in SystemVerilog
By Vyom Parikh, eInfochips
Abstract
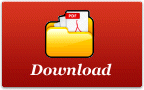
In the current era of machine learning and artificial intelligence, accelerator based SoCs have more complex processing of data and those circuits have software and design verification cycles. These designs also come with software complexity. As a uniform approach, there is always a need for a reference model or a data processing unit as a standard benchmark that is used across all the verticals (functional verification, FPGA, software testing, lab testing, and validation). This is to ensure that the verification cycle/all verticals are completed successfully.
In short, whenever there is data processing, transformation, or manipulation of data, and software interaction comes into the picture, the C model/function is the one that can be used in each vertical. As C is the fundamental language for all other languages, the reference model in C can be used everywhere. This article gives the procedure or step-by-step guide to integrating the C model in the UVM Testbench/environment using the SystemVerilog DPI (Direct Programming Interface) feature.
Why the C model?
There are a couple of reasons why to use the C model. The main/important reasons we can consider are as follows:
- Complexity: For the RTL designs, which have computation algorithms, arithmetic processing, and so on, it will be difficult to implement a similar logic using SystemVerilog.
- Reusability: Generally, the software team uses the reference model to verify the specifications. Their reference models are in C/C++/MATLAB. So, if we use the same reference model in the verification, it will be easy from the design point of view if there is any missing part on the specification or if the design is more complex. In addition, the C models are used in the software/lab testing so we can consider it a golden reference.
Blocks: Having the C model as a reference model in general
The blocks with complex functionality have benefits of the reference model in C as it has a vast set of inbuilt functions & libraries. Some of them are:
- DMA/the block where different vectors are tested
- Processor
- Mathematical block
- Signal Processing/Image processing block that performs different transitions on the signal/images like Fast Fourier Transform (FFT), Inverse matrix calculation, and CORDIC (Coordinate Rotation Digital Computer) algorithm.
C model integration in UVM Testbench
C model can be integrated through a SystemVerilog feature - DPI (Direct Programming interface). DPI is an interface between SystemVerilog and a foreign programming language. It consists of two separate layers: the SystemVerilog layer and a foreign language layer. Both sides of DPI are fully isolated. The programming language that is used as the foreign language is transparent and irrelevant to the SystemVerilog side of the interface. The SystemVerilog compiler does not require to analyze the source code written in the foreign language and similarly, the foreign language compiler does not require to analyze the source code written in the SystemVerilog. Different programming languages can be used and supported with the same intact SystemVerilog layer.[1]
Below is a simple example of how to integrate the C model in the UVM Testbench:
Figure 1: Simple C code
Figure 2: Simple SV code to show how to import the C function
In the example (the two snippets above – Figure 1 & Figure 2), the C file has the function example, and this function is integrated via the statement import “DPI-C” context function void example (); - this line imports the function of the C model.
General Practice:
The real challenge is to use the DPI with the input and output arguments. The syntax to use it in the SV-UVM testbench is {import “DPI-C” context <function_declaration_with_argument>}.
- Import declarations can be anywhere a function can be defined [2]
- Within a Verilog module
- Within a SystemVerilog interface
- Within a SystemVerilog package
- Within a SystemVerilog “compilation unit”
- Import declarations must have a prototype of the arguments
- Must exactly match the number of arguments in the C function
- Must specify compatible data types a mentioned in Table1
- The same C function can be imported in multiple locations
- Each prototype must be the same
- A better method is to define one import in a package [2]
To integrate the C model using DPI-C, one must map the equivalent data type in SV at the time of function declaration.
The table below defines the mapping between the basic SystemVerilog data types and the corresponding C types.
SystemVerilog type | C type |
Byte | Char |
Shortint | short int |
Int | Int |
Longint | long long |
Real | Double |
shortreal | Float |
Chandle | void * |
String | const char * |
Bit | unsigned char |
logic/reg | unsigned char |
Table 1 Mapping between SystemVerilog data types and C data types
The input mode arguments of type byte unsigned and shortint unsigned are not equivalent to bit [7:0] or bit [15:0], respectively, because the former is passed as C types of unsigned char and unsigned short and the latter are both passed by reference as svBitVecVal types. A similar lack of equivalence applies to passing such parameters by reference for output and input modes, e.g., byte unsigned is passed as C type unsigned char* while bit [7:0] is passed by reference as svBitVecVal*.[1]
Different Approaches based on the type of C model
There are many different approaches based on the requirement, usage, and type of the C model. Not all are part of this context, but the general approach is mentioned in this publication. Based on the usage/specification/requirement and the structure of the C model, below are the common practices to use the C model in the SV-UVM testbench:
- File dumping and processing – use the file as input and output of the C model
- Pass by value and pass by reference
- Debug file and display – for quick debug
- Standalone and can work on one data at a time
1.File dumping and processing – Use files as input and output of the C model
Let’s say the C model is taking a file as the input with the fixed format of the file and then processes the data and again dumps it to the output file, where input and output file is given by the DPI-C call from the SV file. This is achieved in the following way:
Figure 3: The C model/function having an argument of files as input and output
Figure 4: The SV code corresponding to the C model mentioned in Figure 3
2. Pass by Value and Pass by Reference
The Pass by Value comes into the picture when the C function argues with the data types. The Pass by Reference comes into the picture when the C function argues in terms of pointers.
For the sake of simplicity, let’s say the C function is taking the input without pointers and the output argument is accessed through the pointer as mentioned in the below snippet. In this case, we need to pass the pointer argument as the output of the function from the SV file at the time of importing the C function using the DPI-C call.
Figure 5:The C code/function having the arguments as Pass by Value and Pass by reference
Figure 6: The SV code corresponds to the C code shown in Figure 5
3. Debug file and display – for quick debug
Debug file and debug display (messages) are mainly required for debugging purposes. To use this approach, the C model must support it. If not, we need to update the C model to print the messages that are required for debugging.
For example, if the C model has prints (messages) and it is guarded under the debug_en/info_en bit, then the debug messages will be printed/displayed when debug_en/info_en is 1. Also, the debug messages can be stored in the file. Below is the example of debug displays based on debug_en bit.
Figure 7: The C code indicating the debug functionality
Figure 8: The SV code corresponding to the C code shown in Figure 7
For the separate debug file, the sC model must initialize the memory for the file and needs to handle the file as mentioned in the below snippet:
Figure 9: The C code showing the functionality of the debug file - how to use
Figure 10: The SV code corresponds to the C code shown in Figure 9
In the above snippet, debug_file.txt is the file, which we can use for debugging. The C model prints the debug messages in the “debug_file.txt” file.
4. Standalone and can work on one data at a time
In this approach, only one data is processed at a time. So, if we have 2048 data then 2048 times DPI-C call will be invoked.
Figure 11: The standalone C code which works on one data at a time
Figure 12: The SV code corresponds to the C code shown in Figure 11
General Challenges
While integrating the C model using DPI, the main challenge is to correctly map the compatible data types in the import statement. An incorrect declaration can lead to unpredictable run-time behavior and does not check for type compatibility.[1]
Warnings/Errors: -
- Stack Trace error:- Indicates something is wrong with the memory allocation.
- Lib error:- When the C code is run with the 32-bit library instead of 64, we might see the error stating incompatible libraries.
- Incorrect value return from the C model/function to SV or vice versa:- Indicates that the data type is not compatible and hence giving the value 0.
Automation if any
One can automate the process of declaration of the function in SV (import “DPI-C” context <function_declaration_with_argument>) with the corresponding data type in C so that there is less chance to get the errors as type compatibility can be taken care of by automation.
Conclusion
By using the Direct Programming Interface construct, we can integrate the C model/code/function in the SV/UVM testbench and the same C code can be used at different stages of the verification and validation cycles.
eInfochips has proven excellence in handling turnkey design verification testing, ODCs for semiconductor product companies across the globe. eInfochips (An Arrow Company) has well-defined methodologies that ensure design verification and validation testing effectiveness. We have in-depth expertise in validation and verification testing diverse systems for functional behavior, compliance to specifications, performance and interoperability. We focus on thorough product validation while optimizing the overall regression and execution time.
Abbreviation
- SV – SystemVerilog
- UVM – Universal Verification Methodology
- DPI – Direct Programming Interface
- BFM – Bus Functional Model
- SoC – System on Chip
References
- SystemVerilog Language Reference Manual. IEEE_Std 1800-2017 – IEEE Standard for SystemVerilog – Unified Hardware Design, Specification, and Verification Language
- 2004-SNUG-Europe-presentation_SystemVerilog_DPI_with_SystemC.pdf – Integrating SystemC Models with Verilog Using the SystemVerilog Direct Programming Interface (DPI) by Stuart Sutherland
About the Author
Vyom Parikh
Vyom Parikh, is working as ASIC Verification Technical Lead (Level 1) at eInfochips. He has an industry experience of 5.5 years in ASIC Design Verification and hands-on working experience in IP level functional verification and worked with multiple clients. He is currently working on SoC functional verification.
If you wish to download a copy of this white paper, click here
|
Related Articles
- System Verilog configurable coverage model in an OVM setup - concept of reusability
- A SystemVerilog DPI Framework for Reusable Transaction Level Testing, Debug and Analysis of SoC Designs
- Density Management in Analog Layout Design: Addressing Issues and Ensuring Consistency
- Dimensioning in 3D space: Object Volumetric Measurement by Leveraging Depth Camera-based Reconstruction on NVIDIA Edge devices
- Bandgap Reference (BGR) Circuit Design and Transient Analysis in 90nm VLSI Technology
New Articles
- Discover new Tessent UltraSight-V from Siemens EDA, and accelerate your RISC-V development.
- The Critical Factors of a High-performance Audio Codec - What Chip Designers Need to Know
- Density Management in Analog Layout Design: Addressing Issues and Ensuring Consistency
- Nexus: A Lightweight and Scalable Multi-Agent Framework for Complex Tasks Automation
- How the Ability to Manage Register Specifications Helps You Create More Competitive Products
Most Popular
![]() |
E-mail This Article | ![]() |
![]() |
Printer-Friendly Page |